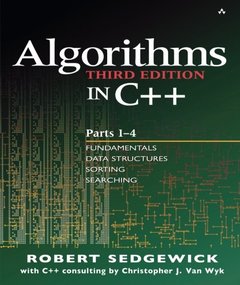
Algorithms in C++, Parts 1-4: Fundamentals, Data Structure, Sorting, Searching, 3/e (Paperback)
內容描述
Description
For this version of Robert Sedgewick's popular textbook on algorithms and data structures, Christopher Van Wyk and Robert Sedgewick have developed new C++ implementations that both express the presented methods in a concise and direct manner, and also provide students with the practical means to test them on real applications.This particular book, Parts 1-4, represents a substantial update of the first half of Sedgewick's complete work. It provides extensive coverage of fundamental data structures and algorithms for sorting, searching, and related applications. The update features expanded coverage of arrays, linked lists, strings, trees, and other basic data structures, and greater emphasis on abstract data types (ADTs), modular programming, object-oriented programming, and C++ classes than in previous editions. It includes over 100 algorithms for sorting, selection, priority queue ADT implementations, and symbol table ADT (searching) implementations, and over 1,000 new exercises to help students learn the properties of algorithms.
Table Of Contents
FUNDAMENTALS. 1. Introduction. Algorithms. A Sample Problem-Connectivity. Union-Find Algorithms. Perspective. Summary of Topics. 2. Principles of Algorithm Analysis. Implementation and Empirical Analysis. Analysis of Algorithms. Growth of Functions. Big-Oh Notation. Basic Recurrences. Examples of Algorithm Analysis. Guarantees, Predictions, and Limitations. DATA STRUCTURES. 3. Elementary Data Structures. Building Blocks. Arrays. Linked Lists. Elementary List Processing. Memory Allocation for Lists. Strings. Compound Data Structures. 4. Abstract Data Types. Abstract Objects and Collections of Objects. Pushdown Stack ADT. Examples of Stack ADT Clients. Stack ADT Implementations. Creation of a New ADT. FIFO Queues and Generalized Queues. Duplicate and Index Items. First-Class ADTs. Application-Based ADT Example. Perspective. 5. Recursion and Trees. Recursive Algorithms. Divide and Conquer. Dynamic Programming. Trees. Mathematical Properties of Trees. Tree Traversal. Recursive Binary-Tree Algorithms. Graph Traversal. Perspective. SORTING. 6. Elementary Sorting Methods. Rules of the Game. Selection Sort. Insertion Sort. Bubble Sort. Performance Characteristics of Elementary Sorts. Shellsort. Sorting Other Types of Data. Index and Pointer Sorting. Sorting Linked Lists. Key-Indexed Counting. 7. Quicksort. The Basic Algorithm. Performance Characteristics of Quicksort. Stack Size. Small Subfiles. Median-of-Three Partitioning. Duplicate Keys. Strings and Vectors. Selection. 8. Merging and Mergesort. Two-Way Merging. Abstract In-Place Merge. Top-Down Mergesort. Improvements to the Basic Algorithm. Bottom-Up Mergesort. Performance Characteristics of Mergesort. Linked-List Implementations of Mergesort. Recursion Revisited. 9. Priority Queues and Heapsort. Elementary Implementations. Heap Data Structure. Algorithms on Heaps. Heapsort. Priority-Queue ADT. Priority Queues for Index Items. Binomial Queues. 10. Radix Sorting. Bits, Bytes, and Words. Binary Quicksort. MSD Radix Sort. Three-Way Radix Quicksort. LSD Radix Sort. Performance Characteristics of Radix Sorts. Sublinear-Time Sorts. 11. Special-Purpose Sorts. Batcher's Odd-Even Mergesort. Sorting Networks. External Sorting. Sort-Merge Implementations. Parallel Sort/Merge. SEARCHING. 12. Symbol Tables and BSTs. Symbol-Table Abstract Data Type. Key-Indexed Search. Sequential Search. Binary Search. Binary Search Trees (BSTs). Performance Characteristics of BSTs. Index Implementations with Symbol Tables. Insertion at the Root in BSTs. BST Implementations of Other ADT Functions. 13. Balanced Trees. Randomized BSTs. Splay BSTs. Top-Down 2-3-4 Trees. Red-Black Trees. Skip Lists. Performance Characteristics. Separate Chaining. Linear Probing. Double Hashing. Dynamic Hash Tables. Perspective. 15. Radix Search. Digital Search Trees. Tries. Patricia Tries. Multiway Tries and TSTs. Text String Index Applications. 16. External Searching. Rules of the Game. Indexed Sequential Access. B Trees. Extendible Hashing. Perspective. Index. 0201350882T04062001